Access Level/Modifiers | Public | Protected | Default | Private |
From the same class | Yes | Yes | Yes | Yes |
From any class in the same package | Yes | Yes | Yes | No |
From a subclass in the same package | Yes | Yes | Yes | No |
From a subclass outside the same package | Yes | Yes, using inheritance | No | No |
From a any non-subclass outside the package | Yes | No | No | No |
Monday, December 28, 2009
Java Access Modifiers/Access Level
Thursday, October 15, 2009
Unique Example of synchronized threads with MouseListener
Below source code is an example of you can manage synchronization of a continuous thread of MouseEntered event with a user defined thread of MouseExited event.
MouseListener interface has abstract methods out of which mouseEntered and mouseExited are the two methods. Suppose, in a JWindow, you have a JProgressBar. When you enter your mouse in the JWindow, it will continuously progress the status of the progress bar. However, if you remove the mouse from the window, progress bar will stop progressing and halts the current status.
Below is the source code. Hope you like it.
import java.awt.*;
import java.awt.event.*;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JProgressBar;
import javax.swing.JWindow;
import javax.swing.SwingConstants;
import javax.swing.UIManager;
import javax.swing.UnsupportedLookAndFeelException;
public class WindowProgressBarThread extends JFrame implements MouseListener, ActionListener, Runnable {
JLabel label;
JButton buttonClick;
JButton buttonClose;
JWindow window;
JProgressBar jProgressBar = new JProgressBar();
private static int statusInt = 0;
private Thread runner;
// A thread that runs as long as the timer
// is running. It sets the text in the label
// to show the elapsed time. This variable is
// non-null when the timer is running and is
// null when it is not running. (This is
// tested in mousePressed().)
// Constants for use with status variable.
private static final int GO = 0, TERMINATE = 1;
private int status;
// This variable is set by mouseEntered() and mouseExited()
// to control the thread. When it's time
// for the thread to end, the value is
// set to TERMINATE.
public WindowProgressBarThread() {
buttonClick = new JButton(" Click On It ");
buttonClose = new JButton("Close");
jProgressBar.setMaximum(100);
jProgressBar.setMinimum(0);
window = new JWindow();
JPanel panel = new JPanel(new GridLayout(1, 0));
JFrame.setDefaultLookAndFeelDecorated(true);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
panel.add(buttonClick);
this.add(panel, BorderLayout.LINE_START);
this.setSize(200,75);
this.pack();
this.setVisible(true);
this.setResizable(false);
this.setLocationRelativeTo(null);
window.addMouseListener(this);
buttonClick.addActionListener(this);
buttonClose.addActionListener(this);
}
// Run method executes while the timer is going.
// Several times a second, it computes the number of
// seconds the thread has been running and displays the
// whole number of seconds on the label. It ends
// when status is set to TERMINATE.
public void run() {
while (true) {
synchronized(this) {
if (status == TERMINATE) {
break;
}
if(statusInt <= 99) { statusInt = statusInt + 1; jProgressBar.setValue(statusInt); jProgressBar.setString(statusInt + "%"); jProgressBar.setStringPainted(true); } } waitDelay(100); } } synchronized void waitDelay(int milliseconds) { // Pause for the specified number of milliseconds OR // until the notify() method is called by some other thread. // (From Section 7.5 of the text.) try { wait(milliseconds); } catch (InterruptedException e) { } } public void mousePressed(MouseEvent evt) { } public void mouseReleased(MouseEvent evt) { } public void mouseClicked(MouseEvent evt) { } synchronized public void mouseEntered(MouseEvent evt) { status = GO; runner = new Thread(this); runner.start(); // start the runner thread (can call it child thread) } synchronized public void mouseExited(MouseEvent evt) { status = TERMINATE; notify(); // Wake up the main thread so that child thread can terminate quickly. jProgressBar.setValue(statusInt); jProgressBar.setString(statusInt + "%"); jProgressBar.setStringPainted(true); runner = null; // stop the runner thread (can call it child thread) } public void actionPerformed(ActionEvent ae) { if(ae.getSource()==buttonClick) { buttonClick.setEnabled(false); statusInt = 0; jProgressBar.setValue(statusInt); jProgressBar.setString(statusInt+"%"); jProgressBar.setStringPainted(true); window.add(new JLabel("Progress Bar Demo",SwingConstants.CENTER), BorderLayout.NORTH); window.add(jProgressBar, BorderLayout.CENTER); window.add(buttonClose, BorderLayout.SOUTH); window.pack(); window.setBounds(800, 500, 200, 75); window.setVisible(true); } if(ae.getSource()==buttonClose){ buttonClick.setEnabled(true); window.setVisible(false); } } public static void main(String[] args) { WindowProgressBarThread windoeProgressThread = new WindowProgressBarThread(); } }

Simple Ajax Example of UserName and Password Validations
Below example has a parent window, where an Edit Label is there. On click on Edit Label, it will go to a child window and will have provision to enter username and password. The username and password, entered, will be checked with those of parent window, which has been populated from a XML file using Ajax.
Before going into the code, let’s have some basics of Ajax:
AJAX is Asynchronous JavaScript and XML and based on JavaScript and HTTP requests. With AJAX, a JavaScript can communicate directly with the server, with the XMLHttpRequest object. With this object, a JavaScript can trade data with a web server, without reloading the page.
AJAX uses asynchronous data transfer (HTTP requests) between the browser and the web server, allowing web pages to request small bits of information from the server instead of whole pages.
Source Codes:
This example consists of five jsps and one Credentials.xml [in the server directory]
testAjax.jsp, time.jsp, EnterCredentials.jsp, UserName.jsp, Password.jsp
If the username and password matches with the one which are stored in the server, it will automatically check the checkbox in the parent window.
testAjax.jsp
time.jsp
EnterCredentials.jsp
UserName.jsp
Password.jsp
Credentials.xml in the server directory

Java Source Code to find the IP Address of the machine
import java.net.NetworkInterface;
import java.util.Enumeration;
public class ipfind {
ipfind(){
try{
Enumeration e = NetworkInterface.getNetworkInterfaces();
while(e.hasMoreElements()){
NetworkInterface ni = (NetworkInterface)e.nextElement();
Enumeration e1 = ni.getInetAddresses();
while(e1.hasMoreElements()){
InetAddress inetAddress = (InetAddress)e1.nextElement();
System.out.println(" " + inetAddress.getHostAddress());
}
}
}catch(Exception e){
}
}
public static void main(String args[]){
ipfind ipf = new ipfind();
}
}
Wednesday, October 14, 2009
Add Swing Components Dynamically using Array List
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.util.ArrayList;
import java.util.List;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JRootPane;
import javax.swing.JScrollPane;
import javax.swing.JTextField;
import javax.swing.SwingUtilities;
import javax.swing.UIManager;
import javax.swing.UnsupportedLookAndFeelException;
public class ButtonAddDynamic implements ActionListener{
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new ButtonAddDynamic().createAndShowGUI();
}
});
}
private JFrame frame;
private JPanel panel = new JPanel(new GridBagLayout());
private GridBagConstraints constraints = new GridBagConstraints();
private List
private List
private List
private JButton button1 = new JButton("Add Another TextField and Button");
private static int countReport = 0;
String files = null;
int y=2;
protected void createAndShowGUI() {
try {
UIManager.setLookAndFeel("com.sun.java.swing.plaf.windows.WindowsLookAndFeel");
} catch (UnsupportedLookAndFeelException ex) {
ex.printStackTrace();
} catch (InstantiationException ex) {
ex.printStackTrace();
} catch (ClassNotFoundException ex) {
ex.printStackTrace();
} catch (IllegalAccessException ex) {
ex.printStackTrace();
}
String[] labels = { "Locations of the given input" };
for (String label : labels)
addColumn(label);
addRowBelow();
constraints.gridx=1;
constraints.gridy=0;
panel.add(button1,constraints);
frame = new JFrame("Add Button Dynamically");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(new JScrollPane(panel));
frame.setLocationRelativeTo(null);
frame.setResizable(false);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
button1.addActionListener(this);
//Set the default button to button1, so that when return is hit, it will hit the button1
JRootPane root = frame.getRootPane();
root.setDefaultButton(button1);
frame.addWindowListener(new WindowAdapter(){
public void windowClosing(WindowEvent we){
System.exit(0);
}
});
}
private void addColumn(String labelText) {
constraints.gridx = fields.size();
constraints.gridy = 1;
panel.add(new JLabel(labelText), constraints);
constraints.gridy=2;
final JTextField field=new JTextField(40);
field.setEditable(false);
panel.add(field,constraints);
fields.add(field);
//constraints.gridy=3;
constraints.gridx = fields.size() + fieldButton.size();
JButton button = new JButton("OK");
button.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent ae){
JOptionPane.showMessageDialog(null,"Program to add swing components dynamically","HI",1);
}
}
);
panel.add(button,constraints);
fieldButton.add(button);
panel.revalidate(); // redo layout for extra column
}
private void addRowBelow() {
y++;
constraints.gridy=y;
//System.out.println(fields.size());
for (int x=0;x < fields.size();x++) {
constraints.gridx=x;
final JTextField field = new JTextField(40);
field.setEditable(false);
panel.add(field, constraints);
constraints.gridx=x+1;
JButton button = new JButton("OK");
button.addActionListener(new ActionListener()
{ public void actionPerformed(ActionEvent ae){
JOptionPane.showMessageDialog(null,"Program to add swing components dynamically","HI",1);
}
}
);
panel.add(button,constraints);
}
}
public void actionPerformed(ActionEvent ae){
if("Add Another TextField and Button".equals(ae.getActionCommand())) {
addRowBelow();
frame.pack();
frame.setLocationRelativeTo(null);
}
}
}

Java Source Code – Slowly Closing the Window of a JFrame – Tricks
import java.awt.event.WindowEvent;
import javax.swing.JFrame;
public class SlowClose {
public SlowClose() {
final JFrame jFrame = new JFrame();
jFrame.setSize(500,350);
jFrame.setVisible(true);
jFrame.setResizable(false);
jFrame.setLocationRelativeTo(null);
jFrame.setTitle("SlowClose");
jFrame.addWindowListener(new WindowAdapter(){
public void windowClosing(WindowEvent we){
int x = 500;
int y = 350;
int d = 10;
while(x > 100 && y > 50){
x = x-d;
y = y-d;
jFrame.setSize(x,y);
System.out.println("X = " + x + "Y = " + y);
try{
Thread.sleep(10000);
}catch(InterruptedException ie){
ie.printStackTrace();
}
if(x <= 100 y <= 50){
System.exit(0);
}
}
}
});
}
public static void main(String args[]) {
SlowClose slowClose = new SlowClose();
}
}
Nobel Winners - 2009
Charles K. Kao – for groundbreaking achievements concerning the transmission of light in fibers for optical communication - China
Willard S. Boyle – for the invention of an imaging semiconductor circuit – the CCD sensor - USA
George E. Smith – for the invention of an imaging semiconductor circuit – the CCD sensor - USA
Chemistry [for studies of the structure and function of the ribosome]
Venkatraman Ramakrishnan – UK
Thomas A. Steitz - USA
Ada E. Yonath – Israel
Physiology or Medicine [for the discovery of how chromosomes are protected by telomeres and the enzyme telomerase]
Elizabeth H. Blackburn - USA
Carol W. Greider - USA
Jack W. Szostak - USA
Literature[ who, with the concentration of poetry and the frankness of prose, depicts the landscape of the dispossessed]
Herta Müller – Germany
Peace – [for his extraordinary efforts to strengthen international diplomacy and cooperation between peoples]
Barrack Obama – USA
The Sveriges Riksbank Prize in Economic Sciences in Memory of Alfred Nobel
Elinor Ostrom - for her analysis of economic governance, especially the commons - USA
Oliver E. Williamson - for his analysis of economic governance, especially the boundaries of the firm - USA
[Source:nobelprize.org]
Saturday, October 10, 2009
SSL Implementation – Java Source Code
This article will help you to implement one way SSL and two way SSL using Java.
Before proceeding into the codes, I would like to explain few terms and concepts which will help you to understand the code in a better way.
Using certificates for privacy and security
You can use certificates to protect your personally identifiable information on the Internet and to protect your computer from unsafe software. A certificate is a statement verifying the identity of a person or the security of a Web site.
Internet Explorer uses two different types of certificates:
A personal certificate is a verification that you are who you say you are. This information is used when you send personal information over the Internet to a Web site that requires a certificate verifying your identity. You can control the use of your identity by having a private key on your computer. When used with e-mail programs, security certificates with private keys are also known as "digital IDs".
A Web site certificate states that a specific Web site is secure and genuine. It ensures that no other Web site can assume the identity of the original secure site. When you are sending personal information over the Internet, it is a good idea to check the certificate of the Web site you are using to make sure that it will protect your personally identifiable information. When you are downloading software from a Web site, you can use certificates to verify that the software is coming from a known, reliable source.
[Source: Microsoft Internet Explorer Help]
How do security certificates work?
A security certificate, whether it is a personal certificate or a Web site certificate, associates an identity with a public key. Only the owner of the certificate knows the corresponding private key. The private key allows the owner to make a digital signature or decrypt information encrypted with the corresponding public key. When you send your certificate to other people, you are actually giving them your public key, so they can send you encrypted information that only you can decrypt and read with your private key.
The digital signature component of a security certificate is your electronic identity card. The digital signature tells the recipient that the information actually came from you and has not been forged or tampered with.
Before you can start sending encrypted or digitally signed information, you must obtain a certificate and set up Internet Explorer to use it. When you visit a secure Web site (one whose address starts with https), the site automatically sends you its certificate.
[Source: Microsoft Internet Explorer Help]
KeyStore and TrustStore
A keystore contains a private key. You only need this if you are a server, or if the server requires client authentication.
A truststore contains CA certifcates to trust. If your server's certificate is signed by a recognized CA, the default truststore will already trust it (because it already trusts trustworthy CAs), so you don't need to build your own, or to add anything to the one from the JRE.
You always need a truststore that points to a file containing trusted certificates, no matter whether you are implementing the server or the client side of the protocol, with one exception. This file is often has a name like cacerts, and by default it may turn out to be a file named cacerts in your jre security directory.
You may or may not need a keystore. The keystore points to a file containing private key material. You need a keystore if 1) you are implementing the server side of the protocol, or 2) you are implementing the client side and you need to authenticate yourself to the server.
SSL provides you with privacy, integrity, and authentication. That is, the messages are encrypted, tamper-evident, and come from an authenticated identity. Whether that's the identity you want to talk to is another question. So the application has to perform the authorization step, i.e. check the identity against what is expected. You do this by getting the peer certificates out of the SSLSession, usually in a HandshakeCompletedListener, and check that the identity of the server is what you expect. SSL can't do this for you as only the application knows who it expects to talk to. Another way around this is to ship a custom truststore that only contains the server certificate for the correct server, so it won't trust anybody else.
AccessUrlSSL.java
/*
Implementation of one way and two way SSL using Java
You can change the mode of the program from one way SSL to two way SSL by changing the flag "oneWaySSL"
If oneWaySSL = true then OneWaySSL, if false then Two Way SSL.
If you need to give proper URL.
Also you need to set the property of:
1. TrustStore location and TrustStore Password to implement one way ssl.
2. KeyStore Location, KeyStorePassword and TrustStore Location, TrustStorePassword to implement two way ssl.
*/
import java.io.BufferedInputStream;
import java.io.BufferedReader;
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.Date;
import javax.net.ssl.HostnameVerifier;
import javax.net.ssl.HttpsURLConnection;
import javax.net.ssl.SSLSession;
public class AccessUrlSSL{
AccessUrlSSL(){
}
private void doUpload(){
boolean oneWaySSL = false; // One Way SSL [true] and Two Way SSL [false]
if(oneWaySSL){
HostnameVerifier hv = new HostnameVerifier() {
public boolean verify(String urlHostName, SSLSession session) {
System.out.println("Warning: URL Host: "+urlHostName+" vs. "+session.getPeerHost());
return true;
}
};
HttpsURLConnection.setDefaultHostnameVerifier(hv);
}
try {
System.setProperty("https.proxySet","true");
System.setProperty("https.proxyHost","internet proxy address");
System.setProperty("https.proxyPort","8080");
System.setProperty("https.proxyType","4");
if(oneWaySSL){
System.setProperty("javax.net.ssl.trustStore","trustStoreLocation");
System.setProperty("javax.net.ssl.trustStorePassword","passw0rd");
}else{
System.setProperty("java.protocol.handler.pkgs","javax.net.ssl");
System.setProperty("javax.net.ssl.keyStore","keystoreLocation"); //KeyStoreLocation - contains the private keys
System.setProperty("javax.net.ssl.keyStorePassword","passw0rd");
System.setProperty("javax.net.ssl.trustStore","trustStoreLocation"); //TrustStoreLocation - contains the trusted sites
System.setProperty("javax.net.ssl.trustStorePassword","passw0rd");
}
if(oneWaySSL){
URL url = new URL("https","siteLocation",443,"siteFolder");
} else {
URL url = new URL("https","siteLocation",443,"siteFolder");
}
HttpsURLConnection con = (HttpsURLConnection) url.openConnection();
con.setDoOutput(true);
con.setDoInput(true);
con.setRequestMethod("POST");
con.setRequestProperty("Connection", "Keep-Alive");
con.setRequestProperty("Content-Type", "multipart/form-data;boundary=vxvxv");
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String str = "";
boolean startPrint = false;
while ((str = in.readLine()) != null) {
System.out.println(str);
}
in.close();
} catch (MalformedURLException me) {
me.printStackTrace();
} catch (IOException ie) {
ie.printStackTrace();
} catch(Exception e){
e.printStackTrace();
}
}
public static void main(String args[]){
AccessUrlSSL accessUrlSSl = new AccessUrlSSL();
accessUrlSSl.doUpload();
}
}
Suggestions and feedback please.
Thursday, October 8, 2009
One Way SSL and Two Way SSL
Here in this article we are mainly concerned about the transmission of message between a SSL client and SSL server. There are two ways how SSL can be implemented between a client and a server. They are: One Way SSL and Two Way SSL.
The best example of a one way SSL authentication is internet banking sites. Whenever you open those kinds of sites, it will generally ask for a warning. This warning pop up will also show you certificates. These certificates are the authentication of the application which you want to access. If you see, there is a lock sign at the right bottom corner of the status bar of the browser. If you double click on it, you will get all the details of the certificates. The certificates have expiry date and issuing authority details, which will confirm you the identification of the application.
Two Way SSL:
In Two way SSL implementation as its name suggests that not only the client authenticates the server, however server also authenticates to the client. Hence, unlike above, here in both server and client, certificates are present and client application verifies the identity of server application and server application also verifies the identity of the client application.
As said above, here not only the server authenticates to the client, also client authenticates itself to the server, that’s why two way SSL is also referred to as client authentication.
The example of two way SSL would be applications which deals with sensitive and confidential data which is intended for a particular recipients. Thus the client who is having the certificates to authenticate itself to the server will only be able to access the application.
In the two way SSL applications, SSL client initiates a connection to a SSL server and server is set to use two way SSL client authentication. The SSL server presents it certificate [which is a public key of the server] to the client for verification. The SSL client verifies it with the private key store of the server. Then SSL server requests SSL client to send its public key to the SSL server to verify with the private key of the SSL client stored in the SSL server.
Any suggestions would very much be appreciated.
Tuesday, October 6, 2009
Create New Sheets in Excel with Name from A to Z using VBA
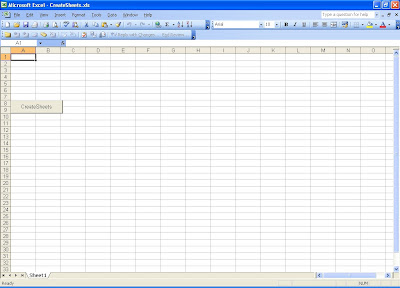
To create sheets:
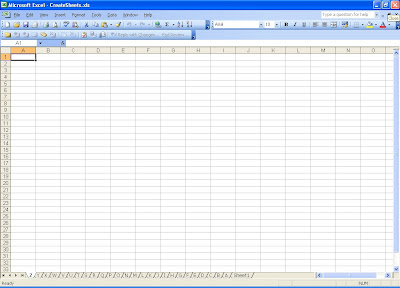
Java Source Code to access the Active Directory Server
An active directory (sometimes referred to as an AD) does a variety of functions including the ability to provide information on objects, helps organize these objects for easy retrieval and access, allows access by end users and administrators and allows the administrator to set security up for the directory.
An active directory can be defined as a hierarchical structure and this structure is usually broken up into three main categories, the resources which might include hardware such as printers, services for end users such as web email servers and objects which are the main
functions of the domain and network.
LDAP is called lightweight because it is a smaller and easier protocol which was derived from the X.500 DAP (Directory Access Protocol) defined in the OSI network protocol stack.
LDAP servers store a hierarchical directory of information. In LDAP parlance, a fully-qualified name for a directory entry is called a Distinguished Name. Unlike DNS (Domain Name Service) FQDN's (Fully Qualified Domain Names), LDAP DN's store the most significant data to the right.
[Source: Internet]
Before going into the code, below are the steps which might reveal the LDAP server name in the MS outlook client.



Below is the code to connect to LDAP server.
ActiveDirectoryDemo.java
import java.util.Hashtable;
import javax.naming.ldap.*;
import javax.naming.directory.*;
import javax.naming.*;
public class ActiveDirectoryDemo {
public static void main(String[] args) {
Hashtable
env.put(Context.SECURITY_PRINCIPAL,"CN=username,OU=companynameOU,DC=company name.com");//User
env.put(Context.SECURITY_CREDENTIALS, "password");//Password
env.put(Context.REFERRAL, "follow");
env.put(Context.INITIAL_CONTEXT_FACTORY, "com.sun.jndi.ldap.LdapCtxFactory");
env.put(Context.PROVIDER_URL, "ldap://servername.Company name.com:port number = 389");
env.put(Context.SECURITY_AUTHENTICATION,"none");
try {
LdapContext context = new InitialLdapContext(env, null);
String base = "";
String filter = "(objectclass=*)";
SearchControls controls = new SearchControls();
controls.setSearchScope(SearchControls.OBJECT_SCOPE);
NamingEnumeration answer = context.search(base, filter, controls);
// ... process attributes ...
while (answer.hasMoreElements()) {
SearchResult sr = (SearchResult)answer.next();
System.out.println("RootDSE: " + sr.getName());
Attributes attrs = sr.getAttributes();
if (attrs != null) {
try {
System.out.println("Naming Context: " + attrs.get("defaultNamingContext").get());
System.out.println("Schema Context: " + attrs.get("schemaNamingContext").get());
System.out.println("DNS: " + attrs.get("dnsHostName").get());
System.out.println("Server Name: " + attrs.get("serverName").get());
}
catch (NullPointerException e) {
e.printStackTrace();
}
}
}
}
catch (NamingException e) {
e.printStackTrace();
}
}
}
Output:

Tuesday, September 1, 2009
To Refresh the JSP page after certain interval of time
Below is the code which can be put into the head tag of the jsp page.
Hope the information might be useful.
Friday, August 21, 2009
Upload a file to a server-Java Source Code [JSP]
To implement upload functionality, we will be using FileUpload and IO packages under common packages of Apache. This will ease out our job of coding this.
Links to download the jar files for the above.
http://commons.apache.org/fileupload/
http://commons.apache.org/io/
We will be creating two JSPs one of which will be the first page which will be asking for the files to browse and upload. After submitting the file, it will give us the details of the file which we have uploaded.
Below are the sample JSPs:
uploadfileToServer.jsp
File Uploading Examples
fileUpload.jsp


Thursday, August 20, 2009
Java Source Code to lock a file
API Source:
http://java.sun.com/javase/6/docs/api/java/nio/channels/FileLock.html
http://java.sun.com/javase/6/docs/api/java/nio/channels/FileChannel.html
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileWriter;
import java.io.RandomAccessFile;
import java.nio.channels.FileChannel;
import java.nio.channels.FileLock;
/**
*
* @author Snehanshu
*/
public class FileLockDemo {
/** Creates a new instance of FileLockDemo */
FileLockDemo() {
}
private void doLockFile() {
try{
File file = new File("./FileLockDemo.txt");
FileLock lock = null;
BufferedWriter bufferedWriter = new BufferedWriter(new FileWriter(file));
bufferedWriter.write("File is being locked");
bufferedWriter.close();
FileChannel channel = new RandomAccessFile(file, "rw").getChannel();
System.out.println("Locking the file");
lock = channel.lock(0, Long.MAX_VALUE, true); // Create a shared lock on the file.
// This section of code will lock the file for 1 mins [60 secs = 60000 milliseconds].
//For those 60 secs, you will not be able to delete or save the file after editing.
System.out.println("Wait for 60 secs for the program to unlock the file");
try {
Thread.sleep(60000);
} catch (InterruptedException ie) {
ie.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
System.out.println("Releasing the lock of the file");
if (lock != null && lock.isValid()) {
lock.release(); // Lock is being released
}
} catch(FileNotFoundException fNFE) {
fNFE.printStackTrace();
} catch(Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
FileLockDemo fileLockDemo = new FileLockDemo();
fileLockDemo.doLockFile();
}
}
Delete a file only when it is closed
try {
Process pc = Runtime.getRuntime().exec("C:/WINDOWS/ServicePackFiles/i386/notepad.exe C:/CheckDemo.txt");
} catch (IOException ex) {
ex.printStackTrace();
}
However I was wondering, if we need to open the file in temporary mode; meaning when the file is closed, it should be automatically deleted.
The waitFor() method of the Process class will do the trick.
The API describes the method like below:
causes the current thread to wait, if necessary, until the process represented by this Process object has terminated. This method returns immediately if the subprocess has already terminated. If the subprocess has not yet terminated, the calling thread will be blocked until the subprocess exits.
[Source: http://java.sun.com/javase/6/docs/api/java/lang/Process.html]
The code is like below:
import java.io.File;
import java.io.IOException;
public class Test {
public static void main(String[] args) throws Exception {
try {
Process pc = Runtime.getRuntime().exec("C:/WINDOWS/ServicePackFiles/i386/notepad.exe C:/CheckDemo.txt");
try {
pc.waitFor();
} catch (InterruptedException ex) {
ex.printStackTrace();
}
File file = new File("C:/CheckDemo.txt");
file.delete();
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
Sunday, August 16, 2009
JcheckBox – Select Any of the checkbox
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import javax.swing.JCheckBox;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class SwingCheckBoxDemo implements ActionListener {
JCheckBox FZYamaha;
JCheckBox XCD135Bajaj;
JCheckBox StunnerHero;
JCheckBox Avenger;
public SwingCheckBoxDemo() {
JFrame.setDefaultLookAndFeelDecorated(true);
JFrame frame = new JFrame("SwingCheckBoxDemo");
frame.setLayout(new BorderLayout());
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
FZYamaha = new JCheckBox("FZ Yamaha");
FZYamaha.setMnemonic(KeyEvent.VK_C);
FZYamaha.setSelected(true);
XCD135Bajaj = new JCheckBox("XCD 135 Bajaj");
XCD135Bajaj.setMnemonic(KeyEvent.VK_G);
XCD135Bajaj.setSelected(false);
StunnerHero = new JCheckBox("Stunner");
StunnerHero.setMnemonic(KeyEvent.VK_H);
StunnerHero.setSelected(false);
Avenger = new JCheckBox("Avenger");
Avenger.setMnemonic(KeyEvent.VK_T);
Avenger.setSelected(false);
/*Put the check boxes in a column in a panel*/
JPanel checkPanel = new JPanel(new GridLayout(0, 1));
checkPanel.add(FZYamaha);
checkPanel.add(XCD135Bajaj);
checkPanel.add(StunnerHero);
checkPanel.add(Avenger);
frame.add(checkPanel, BorderLayout.LINE_START);
frame.pack();
frame.setSize(300,100);
frame.setLocationRelativeTo(null);
frame.setVisible(true);
FZYamaha.addActionListener(this);
XCD135Bajaj.addActionListener(this);
StunnerHero.addActionListener(this);
Avenger.addActionListener(this);
}
public void actionPerformed(ActionEvent ae) {
if(ae.getSource() == FZYamaha) {
System.out.println("Yamaha");
FZYamaha.setSelected(true);
XCD135Bajaj.setSelected(false);
StunnerHero.setSelected(false);
Avenger.setSelected(false);
}
if(ae.getSource() == XCD135Bajaj) {
System.out.println("XCD135Bajaj");
FZYamaha.setSelected(false);
XCD135Bajaj.setSelected(true);
StunnerHero.setSelected(false);
Avenger.setSelected(false);
}
if(ae.getSource() == StunnerHero) {
System.out.println("StunnerHero");
FZYamaha.setSelected(false);
XCD135Bajaj.setSelected(false);
StunnerHero.setSelected(true);
Avenger.setSelected(false);
}
if(ae.getSource() == Avenger) {
System.out.println("Avenger");
FZYamaha.setSelected(false);
XCD135Bajaj.setSelected(false);
StunnerHero.setSelected(false);
Avenger.setSelected(true);
}
}
public static void main(String[] args) {
SwingCheckBoxDemo swingCheckBoxDemo = new SwingCheckBoxDemo();
}
}

Thursday, August 13, 2009
JCheckBox - Java Source Code
import java.awt.BorderLayout;
import java.awt.GridLayout;
import java.awt.event.KeyEvent;
import javax.swing.JCheckBox;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class JCheckBoxDemo {
JCheckBox FZYamaha;
JCheckBox XCD135Bajaj;
JCheckBox StunnerHero;
JCheckBox Avenger;
JCheckBoxDemo() {
JFrame.setDefaultLookAndFeelDecorated(true);
JFrame frame = new JFrame("SwingCheckBoxDemo");
frame.setLayout(new BorderLayout());
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
FZYamaha = new JCheckBox("FZ Yamaha");
FZYamaha.setMnemonic(KeyEvent.VK_C);
FZYamaha.setSelected(true);
XCD135Bajaj = new JCheckBox("XCD 135 Bajaj");
XCD135Bajaj.setMnemonic(KeyEvent.VK_G);
XCD135Bajaj.setSelected(true);
StunnerHero = new JCheckBox("Stunner");
StunnerHero.setMnemonic(KeyEvent.VK_H);
StunnerHero.setSelected(true);
Avenger = new JCheckBox("Avenger");
Avenger.setMnemonic(KeyEvent.VK_T);
Avenger.setSelected(true);
/*Put the check boxes in a column in a panel*/
JPanel checkPanel = new JPanel(new GridLayout(0, 1));
checkPanel.add(FZYamaha);
checkPanel.add(XCD135Bajaj);
checkPanel.add(StunnerHero);
checkPanel.add(Avenger);
frame.add(checkPanel, BorderLayout.LINE_START);
frame.pack();
frame.setSize(300,100);
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
public static void main(String[] args) {
JCheckBoxDemo checkBoxDemo = new JCheckBoxDemo();
}
}

Tuesday, August 11, 2009
Include an addition Tag in XML – Java Source Code
Company.xml [Input]

/*
* AppendChild.java
*/
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.w3c.dom.Text;
import javax.xml.transform.Result;
import javax.xml.transform.Source;
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerException;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.TransformerFactoryConfigurationError;
import javax.xml.transform.dom.DOMSource;
import javax.xml.transform.stream.StreamResult;
/**
*
* @author Snehanshu Chatterjee
*/
public class AppendChild {
/** Creates a new instance of AppendChild */
public AppendChild() {
}
private void appendChildIntoXML(){
}
public static void main(String[] args) {
try{
File xmlDocument = new File("Company.xml");
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document document = builder.parse(xmlDocument);
insertNode(document,xmlDocument);
}catch(Exception e){
e.printStackTrace();
}
}
private static void insertNode(Document doc,File fileDoc){
Node parentNode = doc.getParentNode();
Node officeNode = doc.getElementsByTagName("employee").item(0);
Node telChild = doc.getElementsByTagName("telephone").item(0);
Node addressNode = (Node) doc.createElement("Address");
Node newChild = officeNode.appendChild(addressNode);
officeNode.insertBefore(newChild,telChild);
try {
// create DOMSource for source XML document
Source xmlSource = new DOMSource(doc);
// create StreamResult for transformation result
Result result = new StreamResult(new FileOutputStream(fileDoc));
// create TransformerFactory
TransformerFactory transformerFactory = TransformerFactory.newInstance();
// create Transformer for transformation
Transformer transformer = transformerFactory.newTransformer();
transformer.setOutputProperty("indent", "yes");
// transform and deliver content to client
transformer.transform(xmlSource, result);
} catch (TransformerFactoryConfigurationError factoryError) {
// handle exception creating TransformerFactory
System.err.println("Error creating " + "TransformerFactory");
factoryError.printStackTrace();
}catch (TransformerException transformerError) {
System.err.println("Error transforming document");
transformerError.printStackTrace();
} catch (IOException ioException) {
ioException.printStackTrace();
}
}
}
Run:
set classpath=.
javac AppendChild.java
java AppendChild
pause
Company.XML [Output]:

JMenu – Java Source Code
import java.io.IOException;
import javax.swing.JFrame;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import java.awt.event.ActionListener;
import javax.swing.JOptionPane;
public class ExploreMenu implements ActionListener {
ExploreMenu() {
JFrame frame = new JFrame("Explore Menu");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(300,300);
frame.setResizable(false);
frame.setLocationRelativeTo(null);
// Create the menu bar
JMenuBar menuBar = new JMenuBar();
// Create a menu
JMenu menu = new JMenu("File");
menuBar.add(menu);
// Create a menu item
JMenuItem item = new JMenuItem("Windows");
menu.add(item);
// Install the menu bar in the frame
frame.setJMenuBar(menuBar);
frame.setVisible(true);
item.addActionListener(this);
}
public void actionPerformed(ActionEvent ae) {
if(ae.getActionCommand().equals("Windows")) {
try {
Runtime.getRuntime().exec("explorer C:\\WINDOWS");
}catch(IOException ie){
ie.printStackTrace();
} catch(Exception e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) {
ExploreMenu exploreMenu = new ExploreMenu();
}

Putting the message into IBM Websphere MQ
host=hostname where MQ is installed; localhost if installed locally
port=port on to which connection is established to the channedl
channel=SYSTEM.DEF.SVRCONN
queueManagerName=Queue Manager Name
destinationName=Queue Name where the message will go
isTopic=false
samplefilelocation=./Company.xml
import com.ibm.msg.client.jms.JmsFactoryFactory;
import java.io.*;
import java.io.FileInputStream;
import java.util.Properties;
import javax.jms.*;
public class MQProducer {
public MQProducer() {
}
private void putMessageIntoMQ() {
String propertiesFile = "./ProducerProperties.properties";
this.readPropertyFile(propertiesFile);
Connection connection = null;
Session session = null;
javax.jms.Destination destination = null;
MessageProducer producer = null;
try {
JmsFactoryFactory ff = JmsFactoryFactory.getInstance("com.ibm.msg.client.wmq");
JmsConnectionFactory cf = ff.createConnectionFactory();
cf.setStringProperty("XMSC_WMQ_HOST_NAME", host);
cf.setIntProperty("XMSC_WMQ_PORT", port);
cf.setStringProperty("XMSC_WMQ_CHANNEL", channel);
cf.setIntProperty("XMSC_WMQ_CONNECTION_MODE", 1);
cf.setStringProperty("XMSC_WMQ_QUEUE_MANAGER", queueManagerName);
cf.setStringProperty("XMSC_USERID","username"); //username to connect to MQ
cf.setStringProperty("XMSC_PASSWORD","password"); // password to connect to MQ
connection = cf.createConnection();
session = connection.createSession(false, 1);
if(isTopic)
destination = session.createTopic(destinationName);
else
destination = session.createQueue(destinationName);
producer = session.createProducer(destination);
long uniqueNumber = System.currentTimeMillis() % 1000L;
TextMessage message = session.createTextMessage();
message.setText(sampleFile);
connection.start();
producer.send(message);
System.out.println((new StringBuilder()).append("Sent message:\n").append(message).toString());
recordSuccess();
} catch(JMSException jmsex) {
recordFailure(jmsex);
} finally {
if(producer != null)
try {
producer.close();
} catch(JMSException jmsex) {
System.out.println("Producer could not be closed.");
recordFailure(jmsex);
}
if(session != null)
try {
session.close();
} catch(JMSException jmsex) {
System.out.println("Session could not be closed.");
recordFailure(jmsex);
}
if(connection != null)
try {
connection.close();
} catch(JMSException jmsex) {
System.out.println("Connection could not be closed.");
recordFailure(jmsex);
}
}
System.exit(status);
}
private static void processJMSException(JMSException jmsex) {
System.out.println(jmsex);
Throwable innerException = jmsex.getLinkedException();
if(innerException != null)
System.out.println("Inner exception(s):");
for(; innerException != null; innerException = innerException.getCause())
System.out.println(innerException);
}
private static void recordSuccess() {
System.out.println("SUCCESS");
status = 0;
}
private static void recordFailure(Exception ex) {
if(ex != null)
if(ex instanceof JMSException)
processJMSException((JMSException)ex);
else
System.out.println(ex);
System.out.println("FAILURE");
status = -1;
}
private static String host;
private static int port;
private static String channel;
private static String queueManagerName;
private static String destinationName;
private static boolean isTopic;
private static int status = 1;
private static String sampleFile;
private void readPropertyFile(String fileName) { // reading from the property file
try {
Properties mqProperties = new Properties();
FileInputStream fileInputStream = new FileInputStream(fileName);
mqProperties.load(fileInputStream);
host = mqProperties.getProperty("host");
port = Integer.parseInt(mqProperties.getProperty("port"));
channel = mqProperties.getProperty("channel");
queueManagerName = mqProperties.getProperty("queueManagerName");
destinationName = mqProperties.getProperty("destinationName");
isTopic = Boolean.parseBoolean(mqProperties.getProperty("isTopic"));
sampleFile = mqProperties.getProperty("samplefilelocation");
fileInputStream.close();
} catch (Exception exp) {
exp.printStackTrace();
}
}
public static void main(String args[]) {
MQProducer mqProducer = new MQProducer();
mqProducer.putMessageIntoMQ();
}
}

Getting the Message from MQ – Source Code Java
Today I want to share something new with you which I have learnt few days back.
I was asked to build an application which will consume messages from a messaging queue from remote machine and save into the local machine.
When I saw the MQ, it was IBM websphere MQ in which can manually put the message and test whether our code of getting the message is working or not.
Before walking through the code, it is important to go through the basic terminologies. So please google it and find some useful stuff.
If you happen to install IBM websphere MQ, you will find some of parameters of MQ which we will require to connect to MQ. We will put those parameters into a properties file (ConsumerProperties.properties), so that we can change it without changing the source code.
We assume that the message in the queue will be of XML type and we store the message into an XML file only.
The entries in the properties file will be like the following:
hostName=The host where the MQ server exists; localhost, if locally MQ server is set
qmgrName=Queue Manager Name where channels and the queues are established
port=the port with which the channel is connected
channel=SYSTEM.DEF.SVRCONN
msgFileName=./ConsumedXMLFile
msgDir=./
qName=Queue Name where the message actually comes
Source Code
import com.ibm.mq.*;
import java.util.*;
import java.io.*;
import java.text.SimpleDateFormat;
public class JConsumer {
private MQQueueManager mqQueueManager; // QMGR object
private MQQueue queue; // Queue object
private int openOptionInquire; // Open options
private String hostName; // host name
private String channel; // server connection channel
private String port; // port number on which the QMGR is running
private String qmgrName; // queue manager name
private String qName; // queue name
private String msgFileName; // message file name
private String msgDir;
private static String dateStringPre = new String();
private static String dateStringPost = new String();
private static String dateStringPreTemp = new String();
private static String dateStringPostTemp = new String();
private static boolean statusFlag = false;
private void startDownloading() {
try{
JConsumer MQBrowse = new JConsumer();
MQBrowse.init();
} catch( Exception e) {
e.printStackTrace();
}
}
public void init() {
try{
String fileName = "./ConsumerProperties.properties";
this.readPropertyFile(fileName);
this.mqInit( );
System.out.println("Mq initializing started "+new Date());
} catch( Exception e) {
e.printStackTrace();
}
}
private void mqInit( ) { // Initiation of the MQ parameter
try {
System.out.println("host name : " + hostName+"\n"
+"QMGR name : " + qmgrName+"\n"
+"port number : " + port+"\n"
+"channel : " + channel+"\n"
+"file name : " + msgFileName+"\n"
+"queue name : " + qName+"\n"
+"\n");
mqOperations();
} catch (Exception e) {
e.printStackTrace();
}
}
public void mqOperations() throws Exception { // connect, open, browse, close & disconnects
try {
mqConnect(); // queue manager connection
mqOpen(); // pens the queue & browse
mqClose(); // close the queue
mqDisconnect(); // disconects the queue manager
}
catch (Exception exp) {
exp.printStackTrace();
}
} //mqOperations ends here
private void mqConnect() throws Exception { // Connection to the queue manager
try {
MQEnvironment.hostname = hostName;
MQEnvironment.channel = channel;
MQEnvironment.port = Integer.parseInt(port);
System.out.println("Connecting to ---------- "+hostName + " ---------- " + channel + " ----------- " + port);
mqQueueManager = new MQQueueManager(qmgrName);
System.out.println("Qmgr ---------- " + qmgrName + " connection successfull ");
}
catch ( MQException mqExp) {
System.out.println("Error in connecting to queue manager -- "+qmgrName+" with CC : " + mqExp.completionCode +" RC : " + mqExp.reasonCode);
mqClose();
mqDisconnect();
}
}
private void mqDisconnect() { // disconnect to queue manager
try {
mqQueueManager.disconnect();
System.out.println("Qmgr : " + qmgrName + " disconnect successfull ");
}
catch ( MQException mqExp) {
System.out.println("Error in queue manager disconnect...."+"QMGR Name : " + qmgrName+"CC : " + mqExp.completionCode+"RC : " + mqExp.reasonCode);
}
} // end of mqDisconnect
private void mqOpen() throws MQException {
try {
int openOption = 0;
openOption = MQC.MQOO_BROWSE MQC.MQOO_INPUT_SHARED; // open options for browse & share
queue = mqQueueManager.accessQueue(qName, openOption,qmgrName,null,null);
MQGetMessageOptions getMessageOptions = new MQGetMessageOptions();
getMessageOptions.options = MQC.MQOO_INPUT_AS_Q_DEF;
//MQC.MQGMO_BROWSE_FIRST MQC.MQGMO_WAIT ; //for browsing
getMessageOptions.waitInterval = MQC.MQWI_UNLIMITED;
//MQC.MQWI_UNLIMITED;
// for waiting unlimted times
// waits unlimited
MQMessage message = new MQMessage();
BufferedWriter writer ;
String strMsg;
try {
System.out.println( "waiting for message ... ");
queue.get(message, getMessageOptions);
System.out.println( "Get message sucessfull... ");
byte[] b = new byte[message.getMessageLength()];
message.readFully(b);
strMsg = new String(b);
System.out.println("\n"+strMsg);
// if empty message, close the queue...
if ( strMsg.trim().equals("") ) {
System.out.println("empty message, closing the queue ..." + qName);
}
message.clearMessage();
writer = new BufferedWriter(new FileWriter(msgFileName+"_"+new SimpleDateFormat("yyyyMMddhhmmss").format(new Date())+".xml", true));
writer.write("\n");
writer.write(new String(b));
writer.write("\n");
writer.close();
getMessageOptions.options = MQC.MQOO_INPUT_AS_Q_DEF;
//MQC.MQGMO_BROWSE_NEXT MQC.MQGMO_WAIT ;
} catch (IOException e) {
System.out.println("IOException during GET in mqOpen: " + e.getMessage());
}
} catch ( MQException mqExp) {
System.out.println("Error in opening queue ...."+"Queue Name : " + qName+" CC : " + mqExp.completionCode+" RC : " + mqExp.reasonCode);
mqClose();
mqDisconnect();
}
} //end of mqOpen
private void mqClose() { // close the queue
try {
queue.close();
} catch (MQException mqExp) {
System.out.println("Error in closing queue ...."+"Queue Name : " + qName+" CC : " + mqExp.completionCode+" RC : " + mqExp.reasonCode);
}
}
private void readPropertyFile(String fileName) throws Exception { // reading from the property file
try {
Properties mqProperties = new Properties();
FileInputStream fileInputStream = new FileInputStream(fileName);
mqProperties.load(fileInputStream);
hostName = mqProperties.getProperty("hostName");
qmgrName = mqProperties.getProperty("qmgrName");
port = mqProperties.getProperty("port");
channel = mqProperties.getProperty("channel");
msgFileName = mqProperties.getProperty("msgFileName");
qName = mqProperties.getProperty("qName");
msgDir = mqProperties.getProperty("msgDir");
fileInputStream.close();
} catch (Exception exp) {
exp.printStackTrace();
}
}
public static void main(String args[]){
JConsumer consumer = new JConsumer();
consumer.startDownloading();
}
}
Source Code to log messages in Java Applications
For that we use built in logger jar [log4j-1.2.9.jar - http://www.findjar.com/jar/log4j/jars/log4j-1.2.9.jar.html] which contains all the relevant class of the Logger functionalities. The below simple code logs the information messages, debug messages and the error messages.
Logger API: http://logging.apache.org/log4j/1.2/apidocs/org/apache/log4j/Logger.html
There is one log4jconfig.properties which contains the logger file properties. So we will use another java class to access the properties file.
The entries which I have used in the log4jconfig.properties file are:
log4j.rootLogger=DEBUG,file
log4j.appender.file.layout=org.apache.log4j.HTMLLayout
log4j.appender.file.File=logger.html
log4j.appender.file=org.apache.log4j.RollingFileAppender
log4j.appender.file.MaxFileSize=2000KB
Log4Logger.java
/*
* Log4Logger.java
* Snehanshu Chatterjee
*/
import java.util.Properties;
import org.apache.log4j.Category;
import org.apache.log4j.PropertyConfigurator;
public class Log4Logger extends org.apache.log4j.Logger {
private static Category l=null;
/** Creates a new instance of Log4Logger */
public Log4Logger(String name) {
super(name);
}
static{
try{
Properties logProp=new Properties();
String fName=new String("./log4jconfig.properties");
PropertyConfigurator.configure(fName);
} catch(Exception e) {
e.printStackTrace();
}
}
/**
* This method gets category object and it is static method.
* @param name String.
* @return Category Object with the l field.
*/
public static Category returnLogger(String name) {
l= Log4Logger.getLogger(name);
return l;
}
}
The below code which actually logs any information.
LoggerDemo.java
/*
LoggerDemo.java
Snehanshu Chatterjee
*/
import org.apache.log4j.Category;
public class LoggerDemo {
private static Category OutputCtrl = Log4Logger.returnLogger(LoggerDemo.class.getName());
LoggerDemo() {
}
private void doLog() {
OutputCtrl.info("Info Message");
OutputCtrl.debug("Debug the Program");
OutputCtrl.error("Error Message");
}
public static void main(String args[]) {
LoggerDemo loggerDemo = new LoggerDemo();
loggerDemo.doLog();
}
}
Run the File [Putting java file and jar file in one folder]
set classpath=.;./log4j-1.2.9.jar/
javac LoggerDemo.java
java LoggerDemo
pause
Monday, August 3, 2009
Source code to connect to a Oracle Database – Java
You need to have Oracle Driver [Can be downloaded from http://www.findjar.com/jar/mule/dependencies/maven1/oracle-jdbc/jars/ojdbc14.jar.html], the ip address of the machine where database exists and service identifier of the database. We use 1521 as a default ip address to connect to the database.
/*
* DBConnection.java
*/
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
public class DBConnection {
Connection con;
DBConnection() {
}
private void dbInitiateConnection() {
try {
Class.forName("oracle.jdbc.driver.OracleDriver");
} catch (ClassNotFoundException ex) {
ex.printStackTrace();
} catch(NullPointerException npe) {
npe.printStackTrace();
} catch(Exception e) {
e.printStackTrace();
}
try {
con = DriverManager.getConnection("jdbc:oracle:thin:@ipaddress:1521:serviceidentifierofdatabase","username","password");
con.setAutoCommit(false);
} catch (SQLException se) {
se.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
}
private void getDataFromTable() {
try {
PreparedStatement psd = con.prepareStatement("select empno from emp");
ResultSet rst = psd.executeQuery();
System.out.println("Employee Nos are ");
while(rst.next()){
int empNo = rst.getInt(1);
System.out.println(empNo);
}
rst.close();
psd.close();
} catch (SQLException se) {
se.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
}
private void dbCloseConnection() {
try {
con.close();
} catch(SQLException ex) {
ex.printStackTrace();
} catch(Exception e) {
e.printStackTrace();
}
}
public static void main(String args[]) {
DBConnection dbConnect = new DBConnection();
dbConnect.dbInitiateConnection();
dbConnect.getDataFromTable();
dbConnect.dbCloseConnection();
}
}